Genkit in Node, Building a Weather Service with AI Integration (English)
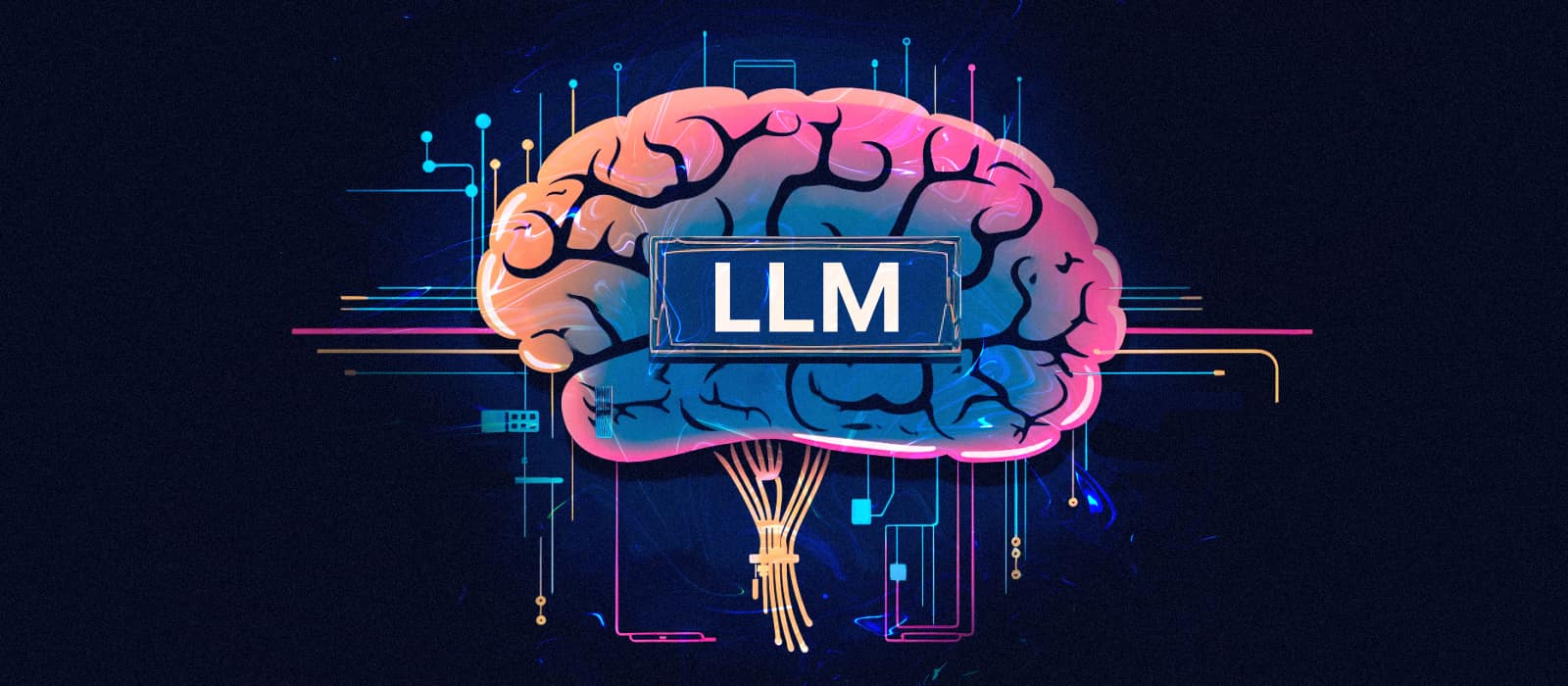
Building a weather service using Genkit in Node.js with AI integration
- Overview
- Prerequisites
- Technical Deep Dive
- Full Code
- Setup & Development
- Dependencies
- Project Configuration
- License
- Resources
- Conclusion
Overview Permalink
This project demonstrates how to build an AI-enhanced weather service using Genkit, TypeScript, OpenWeatherAPI and Github Models. The application showcases modern Node.js patterns and AI integration techniques.
Prerequisites Permalink
Before you begin, ensure you have the following:
- Node.js installed on your machine.
- GitHub account and access token for GitHub APIs.
- An OpenWeatherAPI key for fetching weather data.
- Genkit CLI installed on your machine.
Technical Deep Dive Permalink
AI Configuration Permalink
The core AI setup is initialized with Genkit and GitHub plugin integration. In this case we are going to use the OpenAI o3-mini model:
const ai = genkit({
plugins: [
github({ githubToken: process.env.GITHUB_TOKEN }),
],
model: openAIO3Mini,
});
Weather Tool Implementation Permalink
The application defines a custom weather tool using Zod schema validation:
const getWeather = ai.defineTool(
{
name: 'getWeather',
description: 'Gets the current weather in a given location',
inputSchema: weatherToolInputSchema,
outputSchema: z.string(),
},
async (input) => {
const weather = new OpenWeatherAPI({
key: process.env.OPENWEATHER_API_KEY,
units: "metric"
})
const data = await weather.getCurrent({locationName: input.location});
return `The current weather in ${input.location} is: ${data.weather.temp.cur} Degrees in Celsius`;
}
);
AI Flow Definition Permalink
The service exposes an AI flow that processes weather requests:
const helloFlow = ai.defineFlow(
{
name: 'helloFlow',
inputSchema: z.object({ location: z.string() }),
outputSchema: z.string(),
},
async (input) => {
const response = await ai.generate({
tools: [getWeather],
prompt: `What's the weather in ${input.location}?`
});
return response.text;
}
);
Express Server Configuration Permalink
The application uses the Genkit Express plugin to create an API server:
const app = express({
flows: [helloFlow],
});
Full Code Permalink
The full code for the weather service is as follows:
/* eslint-disable @typescript-eslint/no-explicit-any */
import { genkit, z } from 'genkit';
import { startFlowServer } from '@genkit-ai/express';
import { openAIO3Mini, github } from 'genkitx-github';
import {OpenWeatherAPI } from 'openweather-api-node';
import dotenv from 'dotenv';
dotenv.config();
const ai = genkit({
plugins: [
github({ githubToken: process.env.GITHUB_TOKEN }),
],
model: openAIO3Mini,
});
const weatherToolInputSchema = z.object({
location: z.string().describe('The location to get the current weather for')
});
const getWeather = ai.defineTool(
{
name: 'getWeather',
description: 'Gets the current weather in a given location',
inputSchema: weatherToolInputSchema,
outputSchema: z.string(),
},
async (input) => {
const weather = new OpenWeatherAPI({
key: process.env.OPENWEATHER_API_KEY,
units: "metric"
})
const data = await weather.getCurrent({locationName: input.location});
return `The current weather in ${input.location} is: ${data.weather.temp.cur} Degrees in Celsius`;
}
);
const helloFlow = ai.defineFlow(
{
name: 'helloFlow',
inputSchema: z.object({ location: z.string() }),
outputSchema: z.string(),
},
async (input) => {
const response = await ai.generate({
tools: [getWeather],
prompt: `What's the weather in ${input.location}?`
});
return response.text;
}
);
startFlowServer({
flows: [helloFlow]
});
Setup & Development Permalink
- Install dependencies:
npm install
- Configure environment variables:
GITHUB_TOKEN=your_token OPENWEATHER_API_KEY=your_key
- Start development server:
npm run genkit:start
- To run the project in debug mode and set breakpoints, you can run:
npm run genkit:start:debug
And then launch the debugger in your IDE. See the
.vscode/launch.json
file for the configuration. - If you want to build the project, you can run:
npm run build
- Run the project in production mode:
npm run start:production
Dependencies Permalink
Core Dependencies Permalink
genkit
: ^1.0.5@genkit-ai/express
: ^1.0.5openweather-api-node
: ^3.1.5genkitx-github
: ^1.13.1dotenv
: ^16.4.7
Development Dependencies Permalink
tsx
: ^4.19.2typescript
: ^5.7.2
Project Configuration Permalink
- Uses ES Modules (
"type": "module"
) - TypeScript with
NodeNext
module resolution - Output directory: lib
- Full TypeScript support with type definitions
License Permalink
Apache 2.0
Resources Permalink
Conclusion Permalink
This project demonstrates how to build a weather service using Genkit in Node.js with AI integration. The application showcases modern Node.js patterns and AI integration techniques.
You can find the full code of this example in the GitHub repository
Happy coding!